[Fuente: https://docs.aws.amazon.com/cdk/v2/guide/getting_started.html]
Getting started with the AWS CDK
This topic introduces you to important AWS CDK concepts and describes how to install and configure the AWS CDK. When you’re done, you’ll be ready to create your first AWS CDK app.
Your background
The AWS Cloud Development Kit (AWS CDK) lets you define your cloud infrastructure as code in one of its supported programming languages. It is intended for moderately to highly experienced AWS users.
Ideally, you already have experience with popular AWS services, particularly AWS IAM Identity Center. You might also have experience working with AWS resources programmatically.
Familiarity with AWS CloudFormation is also useful, because the output of an AWS CDK program is an AWS CloudFormation template.
Finally, you should be proficient in the programming language you intend to use with the AWS CDK.
Key concepts
The AWS CDK is designed around a handful of important concepts. We will introduce a few of these here briefly. Follow the links to learn more, or see the Concepts topics in this guide’s Table of Contents.
An AWS CDK app is an application written in TypeScript, JavaScript, Python, Java, C# or Go that uses the AWS CDK to define AWS infrastructure. An app defines one or more stacks. Stacks (equivalent to AWS CloudFormation stacks) contain constructs. Each construct defines one or more concrete AWS resources, such as Amazon S3 buckets, Lambda functions, or Amazon DynamoDB tables.
Constructs (and also stacks and apps) are represented as classes (types) in your programming language of choice. You instantiate constructs within a stack to declare them to AWS, and connect them to each other using well-defined interfaces.
The AWS CDK includes the CDK Toolkit (also called the CLI), a command line tool for working with your AWS CDK apps and stacks. Among other functions, the Toolkit provides the ability to do the following:
- Convert one or more AWS CDK stacks to AWS CloudFormation templates and related assets (a process called synthesis)
- Deploy your stacks to an AWS account and Region
The AWS CDK includes a library of AWS constructs called the AWS Construct Library, organized into various modules. The library contains constructs for each AWS service. The main CDK package is called aws-cdk-lib
, and it contains the majority of the AWS Construct Library. It also contains base classes like Stack
and App
that are used in most CDK applications.
The actual package name of the main CDK package varies by language.
Install | npm install aws-cdk-lib |
---|---|
Import | import * as cdk from 'aws-cdk-lib'; |
Supported programming languages
The AWS CDK has first-class support for TypeScript, JavaScript, Python, Java, C#, and Go. Other JVM and .NET CLR languages may also be used, at least in theory. However, we are unable to offer support for them at this time.
To facilitate supporting so many languages, the AWS CDK is developed in one language (TypeScript). Language bindings are generated for the other languages through the use of a tool called JSII.
We have taken pains to make AWS CDK app development in each language follow that language’s usual conventions. This way, writing AWS CDK apps feels natural, not like writing TypeScript in Python, for example. Take a look at the following examples:
const bucket = new s3.Bucket(this, 'MyBucket', {
bucketName: 'my-bucket',
versioned: true,
websiteRedirect: {hostName: 'aws.amazon.com'}});
The AWS Construct Library is distributed using each language’s standard package management tools, including NPM, PyPi, Maven, and NuGet. There’s even a version of the AWS CDK API Reference for each language.
To help you use the AWS CDK in your favorite language, this guide includes the following topics for supported languages:
- Working with the AWS CDK in TypeScript
- Working with the AWS CDK in JavaScript
- Working with the AWS CDK in Python
- Working with the AWS CDK in Java
- Working with the AWS CDK in C#
- Working with the AWS CDK in Go
TypeScript was the first language supported by the AWS CDK, and much AWS CDK example code is written in TypeScript. This guide includes a topic specifically to show how to adapt TypeScript AWS CDK code for use with the other supported languages. For more information, see Translating TypeScript AWS CDK code to other languages.
Prerequisites
Here’s what you need to install to use the AWS CDK.
All AWS CDK developers, even those working in Python, Java, or C#, need Node.js 14.15.0 or later. All supported languages use the same backend, which runs on Node.js. We recommend a version in active long-term support. Your organization may have a different recommendation.
Other prerequisites depend on the language in which you develop AWS CDK applications and are as follows.
- TypeScript
- TypeScript 3.8 or later (
npm -g install typescript
)
- TypeScript 3.8 or later (
Start an AWS access portal session
Before accessing AWS services, you need an active AWS access portal session for the AWS CDK to use IAM Identity Center authentication to resolve credentials. Depending on your configured session lengths, your access will eventually expire and the AWS CDK will encounter an authentication error. Run the following command in the AWS CLI to sign in to the AWS access portal.
aws sso login
If your SSO token provider configuration is using a named profile instead of the default profile, the command is aws sso login --profile NAME
. Also specify this profile when issuing cdk commands using the –profile option or the AWS_PROFILE
environment variable.
To test if you already have an active session, run the following AWS CLI command.
aws sts get-caller-identity
The response to this command should report the IAM Identity Center account and permission set configured in the shared config
file.
NOTE: If you already have an active AWS access portal session and run aws sso login
, you won’t be required to provide credentials.
The sign in process may prompt you to allow the AWS CLI access to your data. Since the AWS CLI is built on top of the SDK for Python, permission messages may contain variations of the botocore
name.
Install the AWS CDK
Install the AWS CDK Toolkit globally using the following Node Package Manager command.
npm install -g aws-cdk
Run the following command to verify correct installation and print the version number of the AWS CDK.
cdk --version
Note: CDK Toolkit v2 works with your existing CDK v1 projects. However, it can’t initialize new CDK v1 projects. See New prerequisites if you need to be able to do that.
AWS CDK tools
The AWS CDK Toolkit, also known as the Command Line Interface (CLI), is the main tool you use to interact with your AWS CDK app. It executes your code and produces and deploys the AWS CloudFormation templates it generates. It also has deployment, diff, deletion, and troubleshooting capabilities. For more information, see cdk –help or AWS CDK Toolkit (cdk command).
The AWS Toolkit for Visual Studio Code is an open source plug-in for Visual Studio Code that helps you create, debug, and deploy applications on AWS. The toolkit provides an integrated experience for developing AWS CDK applications. It includes the AWS CDK Explorer feature to list your AWS CDK projects and browse the various components of the CDK application. Install the plug-in and learn more about using the AWS CDK Explorer.
CloudFormation
https://aws.amazon.com/cloudformation/
How it works
AWS CloudFormation lets you model, provision, and manage AWS and third-party resources by treating infrastructure as code.
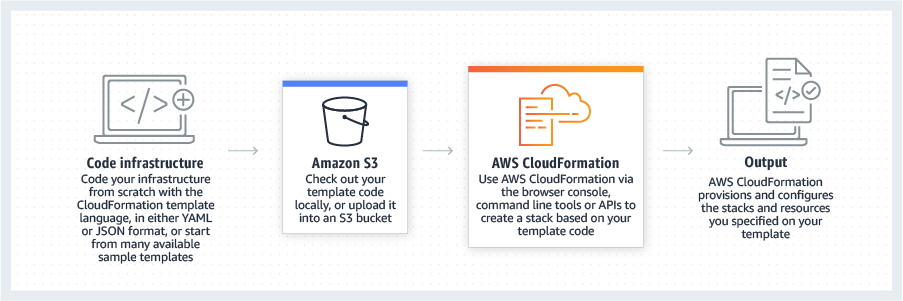
Use cases
Manage infrastructure with DevOps
Automate, test, and deploy infrastructure templates with continuous integration and delivery (CI/CD) automations.
Scale production stacks
Run anything from a single Amazon Elastic Compute Cloud (EC2) instance to a complex multi-region application.
Share best practices
Define an Amazon Virtual Private Cloud (VPC) subnet or provisioning services like AWS OpsWorks or Amazon Elastic Container Service (ECS) with ease.
Stacks
The unit of deployment in the AWS CDK is called a stack. All AWS resources defined within the scope of a stack, either directly or indirectly, are provisioned as a single unit.
Because AWS CDK stacks are implemented through AWS CloudFormation stacks, they have the same limitations as in AWS CloudFormation.
You can define any number of stacks in your AWS CDK app. Any instance of the Stack
construct represents a stack. This can be defined in one of the following ways:
- Directly within the scope of the app, like the
MyFirstStack
example shown previously - Indirectly by any construct within the tree
For example, the following code defines an AWS CDK app with two stacks.
const app = new App();
new MyFirstStack(app, 'stack1');
new MySecondStack(app, 'stack2');
app.synth();
To list all the stacks in an AWS CDK app, run the cdk ls command, which for the previous AWS CDK app would have the following output:
stack1
stack2